Spring에서 제공하는 @Order는 List 형태로 DI 받을때 주입받는 Bean의 우선순위가 필요할때 사용한다. @Order(value = 1), @Order(value = 2), @Order(value = 3).. @Order의 value가 작을수록 우선순위가 높아 앞쪽으로 정렬되고, value가 클수록 우선순위가 낮아 뒷쪽으로 정렬된다. 이 때 주의할 점은 주입받는 N개의 Bean중 일부만 @Order를 사용하면 나머지Bean의 순서는 임의의 순서로 적용된다는 점이다. List중 가장 마지막에 DI 되어야 하는 Bean이 있어서 이 Bean에만 @Order(Ordered.LOWEST_PRECEDENCE) 를 사용했다. Local에서는 이 Bean이 가장 나중에 나와 정상적으로 정렬이 되었는줄 알..
some properties some.url=https://www.some.com 1. @PropertySource - config class @PropertySource("classpath:/my/application/some.properties") or @PropertySources({ @PropertySource("classpath:/my/application/some.properties"), @PropertySource(name="otherProperties", value={"classpath:/my/application/other.properties"}) }) or @PropertySource({"classpath:/my/application/some.properties", "classpath:..
Spring SpEL (Expression Language)은 runtime에 object graph를 query하고 조작하는 강력한 표현언어이다. SpEL 문법은 EL(Unified EL)과 비슷하지만, 메서드 호출과 기본 문자열 템플릿 기능 등 추가적인 기능을 제공한다. OGNL, MVEL, JBoss EL 등의 여러가지 자바 표현언어가 존재하지만 스프링 표현언어는 스프링 포트폴리오의 모든 제품에 걸쳐서 사용할 수 있는 하나의 표현언어를 스프링 커뮤니티에 제공하기 위해서 만들어졌다. SpEL은 기술에 독립적인 API를 기반으로하며 필요에 따라 다른 표현 언어 구현을 통합 할 수 있다. SpEL은 Spring 포트폴리오 내에서 식 평가의 기초로 사용되지만 Spring과 직접 관련이 없으며 독립적으로 사..
1. dependency compile 'com.googlecode.log4jdbc:log4jdbc:1.2' 2. jdbc driver net.sf.log4jdbc.DriverSpy 3. db connection url jdbc:log4jdbc:mysql: 4. logback setting logger('org.springframework.jdbc.core.JdbcTemplate', DEBUG) SQL문만을 로그로 남기며, PreparedStatement일 경우 관련된 argument 값으로 대체된 SQL문이 보여진다. SQL문과 해당 SQL을 실행시키는데 수행된 시간 정보(milliseconds)를 포함한다. ResultSet을 제외한 모든 JDBC 호출 정보를 로그로 남긴다. 많은 양의 로그가 생성되..
비지니스로직을 처리할 때 Exception이 발생하는 경우가 있다. 이 때 에러를 무시할 수 도 있고, 잡아서 다시 처리해야할 때도 있다. 에러를 다시 처리해야할 경우 Spring에서 제공하는 RetryTemplate을 유용하게 사용할 수 있다. Catch 할 Exceptions, Retry Period, Retry Max Count 등을 설정할 수있다. 사용방법은 아래와 같다. //dependency (https://mvnrepository.com/artifact/org.springframework.retry/spring-retry/1.3.0) compile group: 'org.springframework.retry', name: 'spring-retry', version: '1.3.0' //Some..
Spring은 Controller에 Parmeter를 Mapping할 때 Annotation, Debug정보를 참고한다. Annotation은 @PathVarible, @RequestParam, Debug는 Compile할 때 정보를 활용한다. Compile Option은 기본값이 debug=true이지만, 서버에 따라 debug=false로 설정하기도 한다. 이런 상태에서 @PathVarible, @RequestParam이 없는 Controller로 Request되면 Spring은 Parameter Mapping 정보를 찾을 수 없기 때문에 IllegalArgumentException 발생한다. 참고 https://www.slideshare.net/benelog/ss-35627826
출근길에 Out Of Memory Alert을 받았다. 원인은 아침에 kakao plus 친구로 진행한 광고였다. 순간적으로 분당 5,000건으로 Request가 급격하게 증가했다. 하지만 이 서버는 하루중 가장 요청을 받이 받을때에는 5,000건도 원활하게 Request를 처리한다. 그렇다면 왜 Out Of Memory가 발생했을까? Heap Dump를 추출하여 확인해 보았다. 특정 ConcurrentHashMap에서 전체 사용 가능한 Memory의 70% 정도를 사용하고 있었다. 이상했다. 이런 비슷한 상황이 있을것 같아 구글링을 해보았는데 역시나 있었다. 이 상황의 원인과 해결방법은 아래 Blog를 참고했다. 원인은 Controller에서 특정 Page로 Redirect하는 방법이었다. @GetMa..
SomeJpaRepositoryConfig, OtherJpaRepositoryConfig 2개의 Config가 존재한다. 이 경우 SomeJpaRepositoryConfig에 있는 @Primary Annotation으로 인해 OtherJpaRepositoryConfig의 DataSource와 EntityFactory는 SomeJpaRepository의 DataSource와 EntityFactory가 주입된다. @Configuration public class SomeJpaRepositoryConfig { @Bean @Primary public DataSource dataSource() { ... return new DataSource(...); } @Bean @Primary public LocalCont..
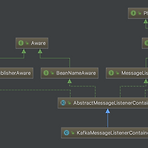
다음과 같은 상황에서 SmartLifecycle Interface를 확장하여 사용한다. require to be started upon ApplicationContext refresh and/or shutdown in a particular order. 특정 순서로 ApplicationContext 새로 고침 및/또는 종료 시 시작되어야 한다. The callback-accepting stop(Runnable) method is useful for objects that have an asynchronous shutdown process. 콜백 수용 정지(Runnable) 방법은 비동기 종료 프로세스가 있는 객체에 유용하다. 직접 구현한 예제 @Service public class SomeKafkaCon..
1. Basic JDBC Template public Integer getTotalCount() { return jdbcTemplate.queryForObject("SELECT COUNT(*) FROM EMPLOYEE", Integer.class); } public Integer modifyEmployeeInformation() { return jdbcTemplate.update("INSERT INTO EMPLOYEE VALUES (?, ?, ?, ?)", 5, "Bill", "Gates", "USA"); } 2. Named Parameter JDBC Template - use preparedStatement public SomeDto getSome(Long id) { String query = "SEL..
- Total
- Today
- Yesterday
- Property
- JPA
- Akka
- spring spel
- guava
- Typesafe Config
- SmartLifecycle
- java EqualsAndHashCode
- Mapping
- docker
- Spring JDBC Template
- Join Table
- Spring Registrar
- Registrar
- java Equals
- DI
- RetryTemplate
- Embedded Mapping
- Criteria
- Sprint RetryTemplate
- 복합키 Mapping
- Spring
- Query DSL
- scikit-learn
- @Primary
- Embeddable Mapping
- Discriminate Mapping
- java generic
- Charles proxy
- JPA Criteria
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 11 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 |